Welcome to my article Coding for Beginners: Your First Python Program Explained. So, you’ve decided to take the plunge into the world of coding—congratulations! But now, the big question: where to start? If you’ve Googled “best programming languages for beginners,” you’ve probably stumbled upon Python more than a few times. Why? Because Python is like the friendly, easygoing neighbor who invites you over for coffee and doesn’t judge you for not knowing how to use the fancy coffee machine. Python’s simple syntax and readability make it an ideal first language, even if the only thing you’ve ever coded before was a grocery list.
In this article, we’re going to walk you through the process of writing your very first Python program. Don’t worry—there will be no confusing jargon, no terrifying technical terms, just a fun, straightforward guide to help you take that first step. We’ll start with something simple (think: “Hello, World!”) and break it down into easy-to-digest chunks. By the end of this tutorial, you’ll have a working program under your belt—and maybe even a little pride in your new skills. Ready to dive in? Let’s roll up our sleeves and get coding!
Access My Proven Blueprint for $50-$100 Daily Income – Watch This FREE Video Now >>>
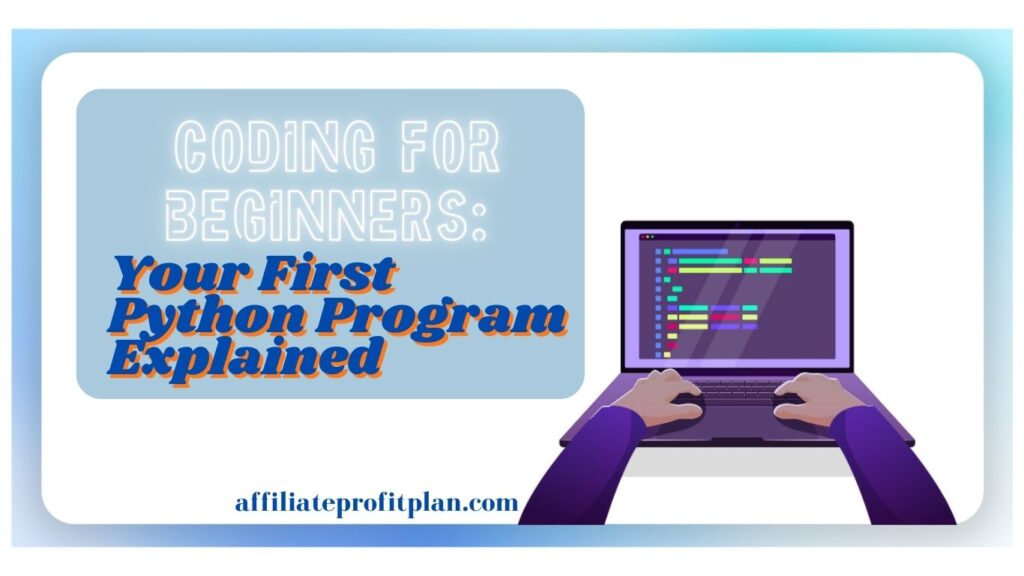
Why Learn Python as Your First Programming Language?
Alright, let’s get one thing straight: learning to code isn’t about conquering a terrifying mountain of cryptic symbols and endless lines of code. It’s about making a new skill set that empowers you to create, solve problems, and (yes, really) have some fun along the way. Enter Python—the friendly neighborhood programming language that’s here to guide you through those early coding jitters with a smile and a cup of coffee. But why exactly is Python the perfect choice for beginners?
First off, Python’s syntax is about as easy to understand as a children’s book. While some programming languages can look like they’ve been designed by caffeinated mathematicians (we’re looking at you, C++), Python is clear and readable, even for someone who’s never seen a line of code before. It’s often said that Python is close to English, which means you won’t need a translator to understand what’s going on. Imagine reading your code aloud, and it makes sense—that’s Python for you! So, instead of getting bogged down with complicated rules, you can focus on learning logic, problem-solving, and building your projects.
Another reason Python is a crowd favorite? It’s everywhere. Whether you’re interested in web development, data science, artificial intelligence, or automation, Python is a jack-of-all-trades. Think of it like that Swiss Army knife you always wanted: it’s versatile, powerful, and can do everything from building websites to analyzing data to teaching machines how to learn. This makes Python not just an awesome first language, but also a long-term ally in your coding journey.
Plus, Python’s vibrant, supportive community is like having a personal coding tutor that’s always there for you. Got a question? There’s a forum for that. Encounter an error? Someone’s probably faced it before and posted a solution online. It’s like joining a global club of people who all love the same language. And the best part? You’re not alone in the struggle to understand it at first—everyone’s been there.
In short, Python’s easy-to-read syntax, versatility, and thriving community make it a no-brainer for beginners. It’s the ideal first language for someone who wants to dip their toes into the vast ocean of programming without getting overwhelmed. Ready to dive in? Your Python adventure starts now!
Setting Up Your Python Environment
Alright, before we dive into writing code, let’s make sure you’ve got everything set up for your coding adventure. Think of setting up your Python environment like getting your workspace ready: You wouldn’t try to write a novel without a notebook (or at least a pen), and you don’t want to write code without the right tools either. But don’t worry—getting everything up and running is easier than you might think, and I promise you won’t have to wrestle with any complex tech jargon. We’ll keep it simple.
Step 1: Installing Python (The Basics)
First things first: you’ll need Python itself. Don’t panic—Python is free to download, and the official website is about as friendly as a Golden Retriever. Here’s what you do:
- Head over to the official Python website: Python.org.
- Download the latest version (Python 3.x) for your operating system. Don’t worry about picking the “right” version—just grab the most recent one and you’re golden.
- Run the installer, and make sure to check the box that says “Add Python to PATH.” This little checkbox will save you from future headaches when you run Python from the command line.
Once that’s done, Python is now a part of your system, ready to roll!
Step 2: Picking an IDE (Your Coding Workspace)
Now, let’s talk about the place where your Python code will come to life: your IDE (Integrated Development Environment). Think of this like your very own creative studio for coding, where you’ll write, edit, and run your programs. There are plenty of options, but if you’re just starting out, I’ve got two great choices for you:
- VS Code: A lightweight but powerful editor that works wonders for Python. It’s like the Swiss Army knife of coding editors. You can download it for free from “Visual Studio Code”
- IDLE: Python comes with its own built-in editor called IDLE, and it’s perfect for beginners. If you’re looking for simplicity, IDLE will do just fine, and it’s already installed when you get Python.
Step 3: Writing Your First Code
Once Python and your IDE are set up, you’re almost ready to start coding! Open your IDE, create a new Python file (make sure it ends with .py), and type this classic beginner code:
python
Copy code
print(“Hello, World!”)
Go ahead and save the file (maybe call it first_program.py), and hit the run button. Voilà—your first Python program is alive and well, and you’ve officially entered the world of coding.
Step 4: Testing It Out
To make sure everything is working smoothly, you can also run Python directly from your terminal (no fancy IDE required). Just open the terminal or command prompt, type python (or python3 depending on your system), and hit enter. If you see a Python version number along with the “>>>” prompt, you’re in Python’s interactive mode, and you’re all set to start typing out simple commands right there.
Bonus Tip: Keep Your Setup Organized
As you continue learning, your projects and Python scripts will pile up. It’s a good habit to keep your coding projects in their own folder (like a little coding “studio” on your computer). This will help you stay organized and make it easier to find your scripts later when you’re looking for that “aha!” moment.
And there you have it! You’ve got Python installed, your IDE set up, and a working environment ready to go. Now, get ready to write some code, because things are about to get interesting!
Writing Your First Python Program
Okay, we’re finally at the fun part—writing your very first Python program! This is where the magic happens. You’ve got Python set up, your IDE is ready, and now it’s time to unleash your inner coding wizard. But don’t worry, you won’t be writing an app to take over the world just yet. We’re going to start small (but mighty) with a classic program that every coder has written at some point: the “Hello, World!” program.
Access My Proven Blueprint for $50-$100 Daily Income – Watch This FREE Video Now >>>
If you’ve ever heard of coding before, you’ve probably come across “Hello, World!”—and for good reason! This little program is like the first step of your coding journey. It’s the equivalent of taking your first steps without tripping over your own feet (hopefully).
Step 1: Open Your IDE and Create a New File
First, open up your favorite IDE (whether it’s VS Code, IDLE, or whatever suits your fancy). Then, create a new Python file. This file will have the .py extension—because Python likes to keep things organized, just like that friend who color-codes their planner.
You could name the file anything, but let’s go with something simple like first_program.py. This will be your canvas, and we’re about to paint some code!
Step 2: Write the Code
Now comes the fun part: writing the actual code. Type in the following line:
python
Copy code
print(“Hello, World!”)
What’s going on here? Glad you asked!
- print: This is a built-in Python function, which is like a helpful assistant who tells Python, “Hey, output this!”
- “Hello, World!”: This is the text you want Python to show you. Notice the quotes? That’s how Python knows that what’s inside is a string (a fancy name for text).
Step 3: Save and Run the Program
Once you’ve written the code, it’s time to run it! Don’t worry, you won’t need to summon any tech gods to make this happen. Just click that “Run” button in your IDE or, if you’re feeling adventurous, head to your terminal (or command prompt) and type:
bash
Copy code
python first_program.py
If everything is working properly, the terminal or the IDE’s output screen will show:
Copy code
Hello, World!
Boom! You’ve just written your first Python program, and it’s as simple as that. It may not look like much yet, but trust me—this is where all the cool stuff starts. You’ve learned the basics: telling Python what to do (with print) and displaying text to the screen.
Step 4: Celebrate (Even if It’s Just a Little)
Go ahead—take a moment to bask in the glow of your very first program. You’ve officially joined the ranks of coders around the world who have proudly printed their first “Hello, World!” Whether it’s a small achievement or a big one in your eyes, it’s a step in the right direction.
Step 5: The Next Steps
Okay, okay, now that you’ve conquered “Hello, World!”, what’s next? Here’s where the real fun begins. The next step is adding a little more interactivity to your program. Why not ask for user input and display it back to them? Here’s an example:
python
Copy code
name = input(“What’s your name? “)
print(f”Hello, {name}!”)
This will prompt the user to type their name, and Python will respond with a personalized greeting. Pretty cool, right? You’re already diving into the basics of interaction, variables, and functions.
Why It’s Important
Writing this first program isn’t just about typing a few lines of code—it’s about understanding how to give instructions to the computer and seeing it respond. It’s your first taste of what coding is all about. And as you write more complex programs, this foundational knowledge will be like the sturdy base of a skyscraper, supporting all the cool stuff you’ll build later on.
So, go ahead and experiment! Change the message inside the print() function. Play with different words and see what happens. Every line of code you write is a mini step toward mastering Python, and every “oops” along the way is just a learning opportunity (trust me, we all make mistakes). You’re officially on your way to becoming a Python pro!
Breaking Down the Code: Understanding the Basics
You’ve written your first Python program—yay! But hold on a second. Before you rush off to write the next big blockbuster app, let’s slow down and take a closer look at the code you just wrote. Understanding the nuts and bolts behind the scenes is what will turn you from a beginner to a confident coder. Trust me, knowing how everything fits together will make coding feel more like solving a puzzle instead of staring at a confusing mess of symbols.
Let’s break down that simple line of code, one piece at a time:
python
Copy code
print(“Hello, World!”)
1. The print Function: Your Code’s Loudspeaker
At the heart of this line is the print() function. This is like the megaphone of Python, shouting whatever message you give it directly to the screen (or console, if we want to get fancy). It’s one of Python’s built-in functions, which means you don’t need to create it yourself—Python already has it ready for you to use.
Think of it like a button you press that tells Python, “Hey, take this message and show it to the user!” It’s one of the most commonly used functions, especially in the beginning, because it’s an easy way to interact with the user and see the results of your code in real-time.
2. The Parentheses () : The Function’s Special Box
In programming, parentheses are pretty important—they’re like the “package” you put your things into. In the case of print(), the parentheses hold the content you want to display. Everything inside these parentheses gets passed into the function for processing.
In this case, the content inside the parentheses is “Hello, World!”. But don’t be fooled by those quotation marks, because they’re not part of the parentheses. They’re just telling Python that the content you’re passing is a string (basically, a fancy term for text). So, inside the parentheses, we have a string—“Hello, World!”—which Python will print for you. You can think of the parentheses as the delivery box, and the string is the gift inside.
3. The Quotation Marks “”: Wrapping It Up in a Cozy Blanket
Those double quotation marks around the text are there for a reason: they mark the beginning and end of a string. Python needs to know where the text begins and where it ends, and the quotation marks are the indicators. Without them, Python would have no idea that “Hello, World!” is a string, and it would probably throw an error at you faster than you can say “syntax mistake.”
You can also use single quotation marks ‘ instead of double quotes, like so:
python
Copy code
print(‘Hello, World!’)
Either way, it’s a way to wrap your text up in a neat little package that tells Python, “This is text. Don’t try to do math with it!”
4. The Semicolon (or Lack Thereof)
Here’s an interesting quirk: unlike some programming languages, Python doesn’t require semicolons at the end of every line of code. This is one of the many things that makes Python so user-friendly. In languages like C++ or Java, you’d need a semicolon to tell the computer, “Hey, that’s the end of the line, let’s move on.” But in Python? Nope, it’s one less thing for you to worry about! Python relies on indentation (spaces and tabs) to figure out where code blocks begin and end. So, for now, just know that you’re free from the semicolon struggle.
5. Putting It All Together
Now that we’ve dissected the line of code, let’s put everything back together and see it in action. When you run:
python
Copy code
print(“Hello, World!”)
Here’s what happens:
- Python sees the print() function and knows it needs to output something to the screen.
- It checks inside the parentheses for the string “Hello, World!”.
- Python takes that string and displays it exactly as you asked, outputting it to the screen for you to see.
It’s simple, but it’s powerful. By understanding these small building blocks, you’re already on your way to creating more complex programs and diving deeper into the world of Python. Each piece of code you write will start making more sense, and before you know it, you’ll be able to write programs that do some seriously cool stuff!
Remember, coding is like learning a new language—every time you write a new line of code, you’re expanding your vocabulary. And once you’ve mastered the basics, you’ll be ready to tackle even bigger and more exciting projects.
So, take a deep breath—you’re doing great! And remember, even the most seasoned programmers started with lines like this one. Keep going, and soon enough, you’ll be writing code that’s as smooth as your morning coffee.
Expanding Your First Program: Adding Features
Alright, now that you’ve successfully written your first Python program and understand how it works, it’s time to step things up! Think of your “Hello, World!” program as a cute little starter home—now it’s time to remodel and add some features to make it a more functional and interesting space. After all, a one-line program is just the beginning, and there’s a whole world of possibilities ahead!
So, what exactly can you add to this program to give it some flair? Well, let’s think about a few fun features we can introduce to give it more personality and interactivity.
1. Asking for User Input: Get Personal
What if you could make your program speak directly to the user? Instead of just displaying a static message, let’s ask the user for their name and personalize the greeting! This is a great way to introduce variables and get the user involved.
Here’s how you do it:
python
Copy code
name = input(“What’s your name? “)
print(f”Hello, {name}!”)
Now, this might look a little more complicated, but let’s break it down:
- input() is a function that pauses your program and waits for the user to type something into the console. The text inside the parentheses (like “What’s your name?”) is the prompt that the user will see.
- The name variable is where we store whatever the user types in. In programming, variables are like containers for holding data, and in this case, we’re storing the user’s name in the name container.
- Finally, we use an f-string (f”Hello, {name}!”) to inject whatever the user typed into the message. It’s like putting a name tag on your greeting: “Hello, John!”
Now, instead of just saying “Hello, World!”, your program is engaging in a conversation with your user. Pretty cool, right?
2. Adding More Interactive Features: Numbers and Math
But wait—why stop there? You can make your program even more interactive by working with numbers. Let’s create a small calculator that asks for two numbers and then adds them together. This will introduce you to the concept of data types (like numbers and strings) and operations (like addition).
Here’s the updated code:
python
Copy code
num1 = float(input(“Enter the first number: “))
num2 = float(input(“Enter the second number: “))
sum = num1 + num2
print(f”The sum of {num1} and {num2} is {sum}.”)
- We use float() to convert the user’s input into a number, which allows us to perform mathematical operations. (By default, input() returns everything as a string, but we need numbers to add them together!)
- The sum variable holds the result of adding the two numbers together.
- Finally, the print() function displays the sum in a friendly message.
Now, instead of just saying “Hello!”, your program is being a helpful assistant, adding numbers and showing the result.
3. Introducing Conditional Statements: Let’s Make Decisions
Let’s kick it up another notch by adding some decision-making to your program. How about a program that greets users based on the time of day? You know, a little “Good morning!” if it’s early, or a “Good evening!” if it’s late.
Here’s a version of the program that greets the user based on the time:
python
Copy code
from datetime import datetime
current_time = datetime.now().hour
if current_time < 12:
print(“Good morning!”)
elif current_time < 18:
print(“Good afternoon!”)
else:
print(“Good evening!”)
- We use the datetime module, which allows Python to access the current date and time.
- datetime.now().hour grabs the current hour of the day.
- The if, elif, and else statements are used to make decisions. If the hour is before 12, it prints “Good morning!”, and so on.
Now your program isn’t just printing random greetings—it’s making decisions based on the time, making it feel much more responsive and dynamic.
4. Adding Loops: Keep the Party Going
If you want your program to do something repeatedly, like asking for the user’s name and greeting them over and over, you can use a loop. This allows you to repeat certain parts of your code without writing it again and again. Let’s set up a simple loop that asks the user their name and says hello multiple times.
Here’s how you can do it:
python
Copy code
for _ in range(3):
name = input(“What’s your name? “)
print(f”Hello, {name}!”)
- The for loop runs a block of code a specific number of times. In this case, range(3) means the loop will run three times.
- The underscore _ is a placeholder since we don’t actually need the loop index in this case.
- The loop asks for the user’s name and prints a greeting three times, without needing to write the same lines of code over and over again.
With just this tiny addition, your program is now more efficient and a lot more fun to interact with!
5. Error Handling: When Things Don’t Go According to Plan
Let’s add a little safety net. What if the user enters something that isn’t a number when your program is expecting one? Rather than letting the program crash, we can make it more robust by handling errors with a try and except block.
Here’s how to do it:
python
Copy code
try:
num1 = float(input(“Enter the first number: “))
num2 = float(input(“Enter the second number: “))
sum = num1 + num2
print(f”The sum is {sum}.”)
except ValueError:
print(“Oops! Please enter a valid number.”)
- The try block attempts to execute the code.
- If the user enters something that isn’t a number, the program will throw a ValueError, and instead of crashing, it will jump to the except block, which prints a friendly error message.
By adding error handling, you’re making your program more user-friendly, ensuring that it doesn’t break when users make mistakes (and let’s face it, we all do).
Why Adding Features is Important
Expanding your program with these features helps you build a deeper understanding of how Python works. You’re not just following along with examples—you’re applying the concepts to create something interactive, functional, and, well, much more fun! Plus, learning how to build on your first program lays the foundation for writing more complex projects in the future.
So, keep experimenting! Add new features, try out new ideas, and don’t be afraid to make mistakes. Every new feature you add makes you a more confident coder. And remember, every great program started somewhere simple—just like yours!
Conclusion: What’s Next on Your Python Journey?
Congratulations, you’ve made it through your first Python program! 🎉 You’ve typed out some code, learned the basics, and maybe even added a few fun features. So, what’s next? Should you close your laptop, take a nap, and tell yourself, “I’m a coding expert now”? Well, not quite just yet—but you’re definitely on the right track!
Access My Proven Blueprint for $50-$100 Daily Income – Watch This FREE Video Now >>>
The journey you’ve started is just the beginning. Python, like a vast, exciting world, is full of endless possibilities. Now that you understand the basics, there’s a whole world of advanced topics, libraries, and frameworks that await you. It’s like learning how to drive a car and now being able to explore a whole new city. So, let’s talk about the next steps to take as you continue your Python adventure!
1. Explore Python Libraries and Frameworks
One of Python’s greatest strengths is its vast collection of libraries and frameworks, which allow you to do everything from web development to machine learning without reinventing the wheel. Libraries like NumPy (for scientific computing), Flask (for web development), and Pandas (for data analysis) can save you hours of work and open up new opportunities for creating powerful projects.
2. Dive Deeper into Object-Oriented Programming (OOP)
Now that you’ve got a solid foundation in Python, it’s time to dive deeper into some of its advanced features, like Object-Oriented Programming (OOP). It might sound intimidating, but think of it as building bigger, more complex Lego structures—OOP teaches you how to organize your code into objects and classes, making your programs more efficient, reusable, and scalable.
3. Practice, Practice, Practice!
While learning Python is fun, the key to mastering it is practice. You’ll get better by writing more code and tackling real-world problems. Try building small projects, like a to-do list app or a simple game. If you hit a roadblock (spoiler alert: you will), don’t worry—it’s part of the learning process. Problem-solving is where the magic happens!
4. Join the Python Community
Coding doesn’t have to be a lonely journey. The Python community is huge, friendly, and always ready to help! You can find online forums, groups, and events where you can ask questions, share your projects, or even collaborate with other Python enthusiasts. Who knows? You might end up working on a cool open-source project or making connections that lead to future opportunities.
5. Learn How to Debug
Even the most experienced developers hit bumps in the road (or, let’s be honest, walls of bugs). Learning how to debug effectively is one of the most important skills you’ll develop. Debugging involves systematically finding and fixing errors in your code. You’ll get really good at this as you gain more experience—so when something goes wrong, you’ll be able to say, “No problem, I got this!”
Final Thoughts
Your first Python program is just the beginning of an exciting journey into the world of programming. The skills you’ve learned—like writing basic code, handling user input, and creating simple loops—are the building blocks for more complex and creative projects. With Python, the possibilities are virtually endless, whether you want to build websites, automate tasks, analyze data, or even create a game.
So, what’s next? Well, the world is your Python oyster! Grab your keyboard, continue exploring, and remember that every bug you fix and every new concept you learn brings you one step closer to becoming a Python pro. Keep coding, stay curious, and have fun on your Python journey—because the best is yet to come! 🚀🐍
Thanks a lot for reading my article on “Coding for Beginners: Your First Python Program Explained” till the end. Hope you’ve helped. See you with another article.